public class MainActivity extends AppCompatActivity { private final static int FILECHOOSER_RESULTCODE = 1; ValueCallback<Uri[]> filePathCallbackLocal; private WebView webView; private String url = "http://192.168.1.253:13599/w/index"; private ValueCallback<Uri> mUploadFile; private String mCameraFilePath; public static void logggg(String log) { Log.v("yqb", log); } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { if (requestCode == FILECHOOSER_RESULTCODE) { if (resultCode == RESULT_OK) { // 获取需上传文件成功 if (null == mUploadFile && null == filePathCallbackLocal) { return; } Uri result = (null == data) ? null : data.getData(); if (result == null) { // 从相机获取 File cameraFile = new File(mCameraFilePath); if (cameraFile.exists()) { result = Uri.fromFile(cameraFile); // 扫描相册 if (filePathCallbackLocal != null) { filePathCallbackLocal.onReceiveValue(new Uri[]{result}); filePathCallbackLocal = null; } else { mUploadFile.onReceiveValue(result); mUploadFile = null; } } } else { try { if (mUploadFile != null) { mUploadFile.onReceiveValue(result); mUploadFile = null; } else if (filePathCallbackLocal != null) { filePathCallbackLocal.onReceiveValue(new Uri[]{result}); filePathCallbackLocal = null; } } catch (Exception e) { // MeilaLog.e(TAG, e); } } } else { // 获取需上传文件失败或者取消操作 if (mUploadFile != null) { mUploadFile.onReceiveValue(null); mUploadFile = null; } else if (filePathCallbackLocal != null) { filePathCallbackLocal.onReceiveValue(null); filePathCallbackLocal = null; } } } } @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); webView = (WebView) findViewById(R.id.webview); WebSettings webSettings = webView.getSettings(); webSettings.setJavaScriptEnabled(true); webSettings.setSupportZoom(true); webSettings.setSupportMultipleWindows(false); webSettings.setUseWideViewPort(true); webSettings.setLoadWithOverviewMode(true); //开启storage webSettings.setDomStorageEnabled(true); webSettings.setJavaScriptCanOpenWindowsAutomatically(true); webView.setWebChromeClient(new WebChromeClient() { //The undocumented magic method override //Eclipse will swear at you if you try to put @Override here // For Android 3.0+ public void openFileChooser(ValueCallback<Uri> uploadMsg) { if (mUploadFile != null) { mUploadFile.onReceiveValue(null); } else { mUploadFile = uploadMsg; } logggg("\"openFileChooser(ValueCallback<Uri> uploadMsg)\""); MainActivity.this.startActivityForResult(createDefaultOpenableIntent(), MainActivity.FILECHOOSER_RESULTCODE); } // For Android 3.0+ public void openFileChooser(ValueCallback uploadMsg, String acceptType) { if (mUploadFile != null) { mUploadFile.onReceiveValue(null); } else { mUploadFile = uploadMsg; } logggg("ValueCallback uploadMsg, String acceptType"); MainActivity.this.startActivityForResult(createDefaultOpenableIntent(), MainActivity.FILECHOOSER_RESULTCODE); } //For Android 4.1 public void openFileChooser(ValueCallback<Uri> uploadMsg, String acceptType, String capture) { if (mUploadFile != null) { mUploadFile.onReceiveValue(null); } else { mUploadFile = uploadMsg; } logggg("ValueCallback<Uri> uploadMsg, String acceptType, String captur"); MainActivity.this.startActivityForResult(createDefaultOpenableIntent(), MainActivity.FILECHOOSER_RESULTCODE); } @Override public boolean onShowFileChooser(WebView webView, ValueCallback<Uri[]> filePathCallback, FileChooserParams fileChooserParams) { if (filePathCallbackLocal != null) { filePathCallbackLocal.onReceiveValue(null); } else { filePathCallbackLocal = filePathCallback; } logggg("WebView webView, ValueCallback<Uri[]> filePathCallback, FileChooserParams fileChooserParams"); startActivityForResult(createDefaultOpenableIntent(), MainActivity.FILECHOOSER_RESULTCODE); return true; } }); webView.setWebViewClient(new MyWebViewClient()); webView.loadUrl(url); } private Intent createDefaultOpenableIntent() { // Create and return a chooser with the default OPENABLE // actions including the camera, camcorder and sound // recorder where available. Intent i = new Intent(Intent.ACTION_GET_CONTENT); i.addCategory(Intent.CATEGORY_OPENABLE); i.setType("*/*"); Intent camcorderIntent = new Intent(MediaStore.ACTION_VIDEO_CAPTURE); Intent soundRecorderIntent = new Intent(MediaStore.Audio.Media.RECORD_SOUND_ACTION); Intent chooser = createChooserIntent(createCameraIntent(), soundRecorderIntent); chooser.putExtra(Intent.EXTRA_INTENT, i); chooser.putExtra(Intent.EXTRA_TITLE, "请选择要上传的文件"); return chooser; } private Intent createCameraIntent() { Intent cameraIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); File externalDataDir = Environment.getExternalStoragePublicDirectory( Environment.DIRECTORY_DCIM); File cameraDataDir = new File(externalDataDir.getAbsolutePath() + File.separator + "browser-photos"); cameraDataDir.mkdirs(); mCameraFilePath = cameraDataDir.getAbsolutePath() + File.separator + System.currentTimeMillis() + ".jpg"; cameraIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(new File(mCameraFilePath))); return cameraIntent; } private Intent createChooserIntent(Intent... intents) { Intent chooser = new Intent(Intent.ACTION_CHOOSER); chooser.putExtra(Intent.EXTRA_INITIAL_INTENTS, intents); return chooser; } public class MyWebViewClient extends WebViewClient { @Override public void onPageFinished(WebView view, String url) { super.onPageFinished(view, url); } } }
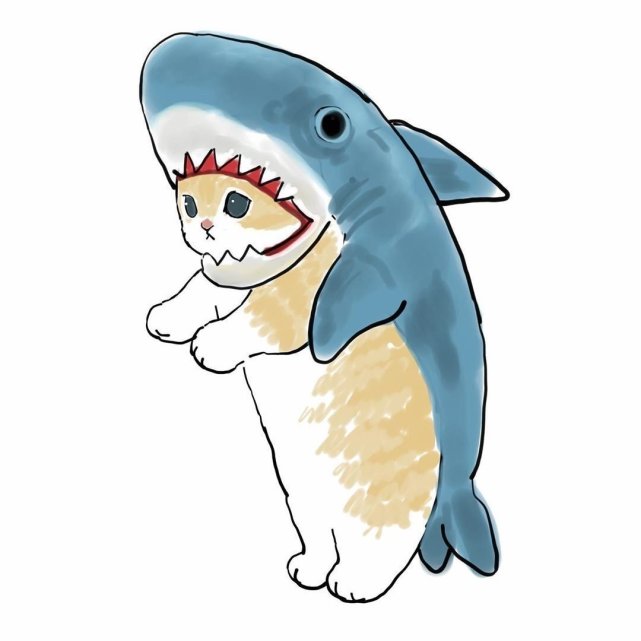